App
The Devprime platform provides a modern software architecture strategy, and the App project is the initial project of the application, allowing connectivity with other components of the architecture.
Exploring the Devprime App
The Devprime App project provides some core files, such as App.cs, App.csproj, appsettings.json, and GlobalUsings.cs, as shown in the image.
These files are responsible for the application startup settings, dependency inversion configuration, initial app.net settings, Swagger, security and, especially, the parameters of each adapter, which are dealt with in detail in the Settings section of the documentation.
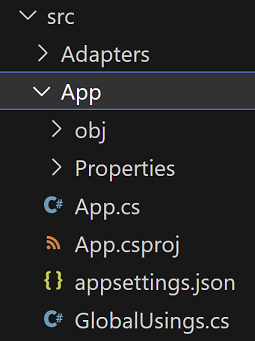
Exploring the file App.cs
The App.cs
file contains the implementations that are fundamental to the initialization of the application and follows a standard ASP.NET processing order criterion. An important point in this context is the creation of the Builder and Run, as well as the dependency injections that connect the entire application architecture project.
the name of the application or microservice is defined at that point in line 23 DpApp(builder).Run("my-microsservices,(app))
and was automatically defined when the new microservice was created using the dp new
command from the Devprime CLI.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
|
// Application Builder
var builder = WebApplication.CreateBuilder(args);
// Adding records for dependency injection.
// This block is modified when you run the
// dp init command to include new classes.
builder.Services.AddScoped<IPaymentRepository, PaymentRepository>();
builder.Services.AddScoped<IPaymentService, PaymentService>();
builder.Services.AddScoped<IPaymentState, PaymentState>();
builder.Services.AddMvc(o =>
{
o.EnableEndpointRouting = false;
});
//Registering Devprime Adapters
builder.Services.AddScoped<IExtensions, Extensions>();
builder.Services.AddScoped<IEventStream, EventStream>();
builder.Services.AddScoped<IEventHandler,
Application.EventHandlers.EventHandler>();
// Application Run
await new DpApp(builder).Run("my-microsservices", (app) =>
{
//The first stage of middleware registration
app.UseRouting(); //Routes
app.UseAuthentication(); //Security
DpApp.UseDevPrimeSwagger(app); //Swagger
app.UseAuthorization();//Security
app.MapControllerRoute(name: "default", pattern:
"{controller=Home}/{action=Index}/{id?}");//Routes
}, (builder) =>
{
//The second stage of middleware registration
DpApp.AddDevPrime(builder.Services);//Accelerators
DpApp.AddDevPrimeSwagger(builder.Services);//Swagger
DpApp.AddDevPrimeSecurity(builder.Services);//Security
});
|
Exploring the file App.csproj
The file App.csproj
brings the dependencies to the other “Adapters” and “Core” projects that are part of the software architecture, as well as to the devprime.stack.app
component of the Devprime Stack, which has intelligent behaviors that serve the context of the “App”.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
|
<Project Sdk="Microsoft.NET.Sdk.Web">
<PropertyGroup>
<TargetFramework>net7.0</TargetFramework>
<Version>1.0.0.0</Version>
</PropertyGroup>
<ItemGroup>
<ProjectReference Include=
"..\Adapters\Services\DevPrime.Services.csproj" />
<ProjectReference Include=
"..\Adapters\State\DevPrime.State.csproj" />
<ProjectReference Include=
"..\Adapters\Extensions\DevPrime.Extensions.csproj" />
<ProjectReference Include=
"..\Adapters\Stream\DevPrime.Stream.csproj" />
<ProjectReference Include=
"..\Adapters\Web\DevPrime.Web.csproj" />
<ProjectReference Include=
"..\Adapters\Observability\DevPrime.Observability.csproj" />
<ProjectReference Include=
"..\Adapters\AppCenter\DevPrime.AppCenter.csproj" />
<ProjectReference Include=
"..\Adapters\HealthCheck\DevPrime.HealthCheck.csproj" />
<ProjectReference Include=
"..\Core\Application\Application.csproj" />
<ProjectReference Include=
"..\Core\Domain\Domain.csproj" />
</ItemGroup>
<ItemGroup>
<PackageReference Include=
"devprime.stack.app" Version="7.0.50" />
</ItemGroup>
</Project>
|
This is a standard implementation, and there is no need to modify the structure unless you want to adjust the following items:
Topic |
Description |
TargetFramework |
.NET version and present in all projects |
Version |
Update the version ID by DevOps |
PackageReference |
Devprime Stack Context Reference |
The dp stack
command, provided by the Devprime CLI, should be used by the developer to automatically update the Devprime reference across all projects.
Exploring the Devprime project GlobalUsings.cs
The C# strategy for globally organizing the namespaces used in the project and allows you to update manually or by using the dp init
from the Devprime CLI, if needed.
1
2
3
4
5
6
7
8
9
10
11
12
13
|
global using DevPrime.Stream;
global using DevPrime.Stack.Foundation.Stream;
global using DevPrime.Stack.Foundation;
global using DevPrime.Stack.App.Load;
global using Microsoft.AspNetCore.Builder;
global using Microsoft.Extensions.DependencyInjection;
global using DevPrime.Extensions;
global using Application.Interfaces.Adapters.Extensions;
global using Application.Interfaces.Adapters.State;
global using Application.Interfaces.Services;
global using Application.Services.Payment;
global using DevPrime.State.Repositories.Payment;
global using DevPrime.State.States;
|
Exploring the file appsettings.json
The Devprime platform provides the possibility of customization through the configuration file, which, in the development environment, is in the Devprime APP project and includes items such as: APP, Web, Stream, Observability, Security, State, Services and Custom.
Here’s a brief example of the appsettings.json
file. You can explore it in detail in the settings section and get more details about the customization parameters.
1
2
3
4
5
6
7
8
9
10
|
{
"DevPrime_App": {},
"DevPrime_Web": {},
"DevPrime_Stream": {},
"DevPrime_Observability": {},
"DevPrime_Security": {},
"DevPrime_Services": {},
"DevPrime_State": {},
"DevPrime_Custom": {}
}
|
Settings |
Details |
APP |
Main Application Parameters |
Web |
Web Adapter Parameters |
Stream |
Adapter Stream Parameters |
Observability |
Adapter Observability Parameters |
Security |
Adapter Security Parameters |
State |
Adapter State Parameters |
Services |
Adapter Services Parameters |
Custom |
Customized parameters for the application |
Explore other related technologies
Last modified April 16, 2024 (2b35fcc8)